Introduction to Tkinter
This lesson will teach you how to use the Tool Kit Interface
tkinter module to create Graphical User Interfaces (GUIs) for people to use your python programs.
Creating Windows
Create a new file called tkinterCode.py to write this program.
Import the tkinter module at the top of the file
import tkinter
Next, we will create a tkinter window with the Tk() function, and set the size of the window with the geometry() function.
import tkinter tk_window = tkinter.Tk() tk_window.geometry("400x300")
The string argument changes the size of the window. The first number is the width of the window in pixels (400), and the second number is the height of the window in pixels (300).
Next, we're going to change the name of the window just like we did with the turtle module. To change it, we'll use the title() function.
import tkinter tk_window = tkinter.Tk() tk_window.geometry("400x300") tk_window.title("GUI")
Now run the program to see what happens. A Tkinter window should appear with the title of
GUI
Press the X button in the top right of the window to close it.
Creating Buttons
Tkinter allows us to add objects called widgets to our application. Examples of widgets are buttons, labels, checkbuttons etc.
You can read about all the different Tkinter widgets here. For now we will look at only few so let's start with buttons.
Adding buttons lets people use your program in different ways. We'll define a function that shows what will happen when the button is pressed, and set the button to display in the window.
First let's define a function that will run whenever we click the button.
We will call the function print_hello() and it will simply print
Helloto the shell window.
import tkinter tk_window = tkinter.Tk() tk_window.geometry("400x300") tk_window.title("GUI") def print_hello(): print("Hello")
Next we create the button using the tkinter Button() method.
This method has two parameters. The first is the text parameter that is assigned a string for what the button will be labeled.
The second is the command parameter which is assigned the name of the function to execute when the button is clicked.
Note that the definition of the button must come after the definition of the function the button calls in the command
import tkinter tk_window = tkinter.Tk() tk_window.geometry("400x300") tk_window.title("GUI") def print_hello(): print("Hello") button = tkinter.Button(text="Say Hello",command=print_hello)
After the button is defined, it can be placed on the screen using the grid() function.
Tkinter widgets are positioned in a table-like structure with columns and rows.
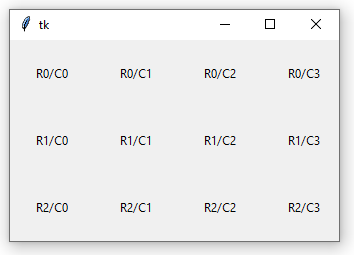
The tkinter grid function takes in a column and a row argument which are used to place the widgets in the grid.
import tkinter tk_window = tkinter.Tk() tk_window.geometry("400x300") tk_window.title("GUI") def print_hello(): print("Hello") button = tkinter.Button(text="Say Hello",command=print_hello) button.grid(column=0,row=1)
Run the program and you should now see a button in the window.
Click the button and look in the Shell window to see what happens.
Creating Labels
Another Tkinter widget we can add is the label widget.
Labels are used to display text or images which are useful for identifying parts of the interface or giving feedback to the user.
We can use the Label() function to create a label and assign a string to the text parameter for what we want the label to say. Once again, we use the grid() function to position the widget.
import tkinter tk_window = tkinter.Tk() tk_window.geometry("400x300") tk_window.title("GUI") def print_hello(): print("Hello") button = tkinter.Button(text="Say Hello",command=print_hello) button.grid(column=0,row=1) label = tkinter.Label(text="Hello There") label.grid(column=0,row=2)
The final window will look like this:
In the next lesson, we'll use the tkinter module to create a rock-paper-scissors game.