First Python Project
This lesson will teach you how to make a python project and use variables.
Creating a New Program
Open the search dialog from the start menu (Windows) or open the search dialog from the top right (MAC) and type IDLE.
Open the program and a window will appear. This window is called the Shell and can be used to write code and display results from our programs.- We will not write code here as the Shell only runs one line of code at a time. Instead we want to write our programs in new files then run all the code at once.
To create a new file select File> New File.
To save the file, select File > Save As...
Save it as firstproject.py to any location. In this video we'll save it to the desktop.
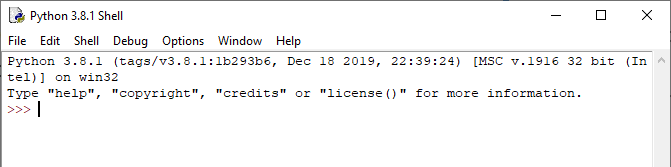
There are some default settings in IDLE you might want to change. You can add line numbers to your code so it is easier to explain to others which line of code you are talking about, and you can disable a window that will appear every time you try to run your program without saving.
To access the settings window in IDLE, go to Options > Configure IDLE then click the Shell/Ed tab.
For the At Start of Run (F5) option, select No Prompt. This will automatically save your code every time you try to run it.
Check the box that says Show line numbers in new windows.
Click Apply to apply your changes. You will not see any line numbers in your IDLE session yet, since the option you checked was just for new files.
To show or hide line numbers for your currently-opened file, click Options > Show Line Numbers.
To change the IDLE Theme to Dark Mode, go to Options > Configure IDLE then click the Highlights tab.
In the Highlight Theme section change IDLE New to IDLE Dark.
To access the settings window in IDLE, go to Options > Configure IDLE then click the Shell/Ed tab.
For the At Start of Run (F5) option, select No Prompt. This will automatically save your code every time you try to run it.
Check the box that says Show line numbers in new windows.
Click Apply to apply your changes. You will not see any line numbers in your IDLE session yet, since the option you checked was just for new files.
To show or hide line numbers for your currently-opened file, click Options > Show Line Numbers.
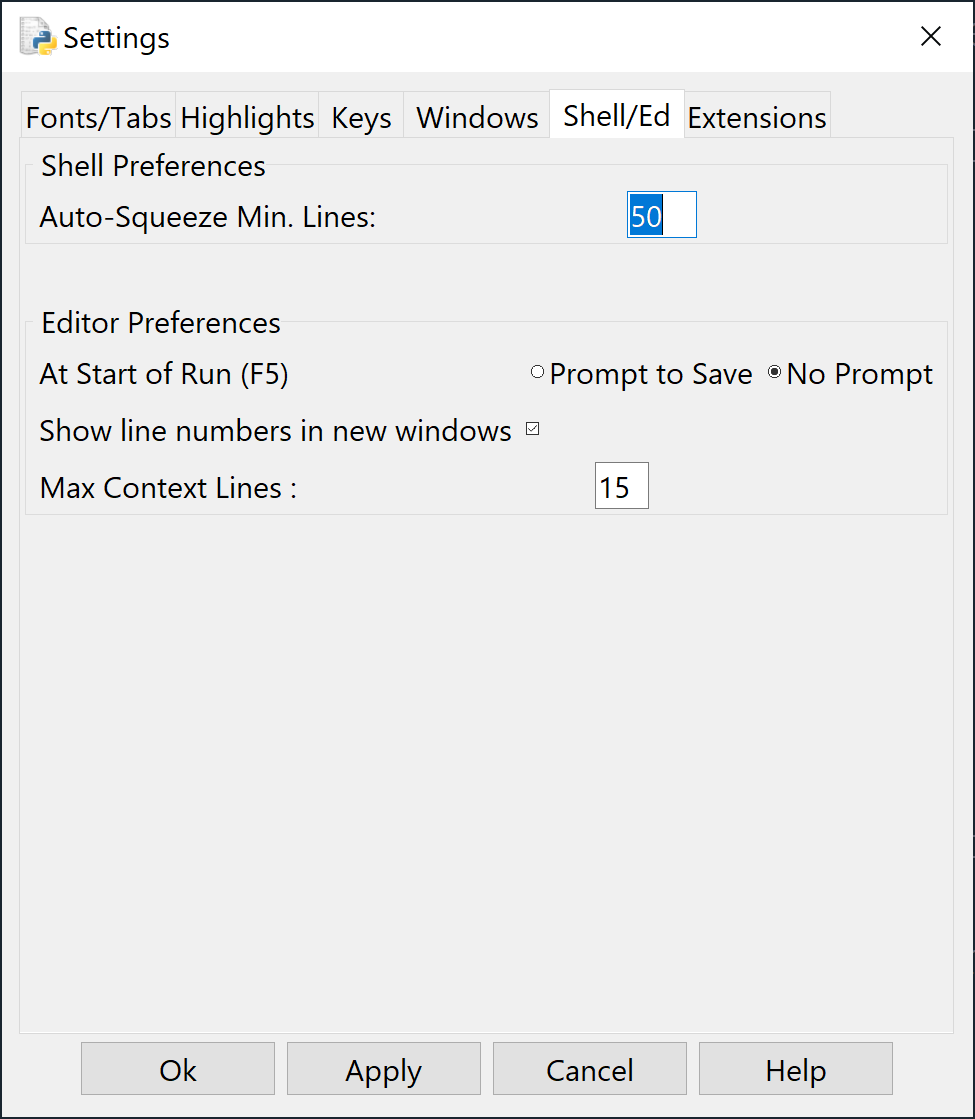
To change the IDLE Theme to Dark Mode, go to Options > Configure IDLE then click the Highlights tab.
In the Highlight Theme section change IDLE New to IDLE Dark.
Creating Variables
- Python has many types of data that are all used for different things.
You do not need to remember all of the data types, but here they are for reference.
Catagory Data Types Text str Numeric int, float, complex Sequence list, tuple, range Mapping dict Set set, frozenset Boolean bool Binary bytes, bytearray, memoryview
Variables assume the data type of the values assigned to them.
For now we will only use: strings (a collection of characters), integers (positive and negative whole numbers) and floats (decimal numbers).
Create a variable by writing the name you want to call it. This is known as the identifier.
Note: the numbers on the left side of the code blocks are to help make them more readable, you shouldn't be writing in those line numbers into your code.
age
You can then assign a value of any data type to the variable using the equals sign (called the assignment operator), followed by the value you want to set it to. The age variable below is now of the data type int with a value of 12.
age = 12
To assign a string to a variable you need to surround it in quotes. E.g. name =David
- If an identifier needs multiple words, try using camel case or underscores.
Camel Case: theWordVariable
Underscores: the_word_variable (typically used in python)
There are some rules to follow when creating identifiers for variables.
1. Identifiers may only contain uppercase letters (A to Z), lowercase letters (a to z), underscores (_), and numeric digits (0 to 9).
2. Identifiers must begin with an underscore, an uppercase letter, or lowercase letter.
3. The identifier must not have any special characters such as @, $, or %. It also cannot contain spaces.
4. The identifier cannot be a keyword. A keyword is an identifier that already exists by default in Python. E.g. print.
5. Identifiers are case sensitive. E.g. The identifier called name is not the same as the identifier called Name.
2. Identifiers must begin with an underscore, an uppercase letter, or lowercase letter.
3. The identifier must not have any special characters such as @, $, or %. It also cannot contain spaces.
4. The identifier cannot be a keyword. A keyword is an identifier that already exists by default in Python. E.g. print.
5. Identifiers are case sensitive. E.g. The identifier called name is not the same as the identifier called Name.
Printing Values
Python has many built-in functions that allow us to do things. We will look at the print function now.
Printing a variable will display it in the Shell window.
To print a value, type print(value)
print(12)
To print a variable, you can type print(variable_name)
Save your file: Save your file after you type code by clicking File > Save or pressing CTRL + S on your keyboard.
Run your program: After you save, you can run your program by clicking Run > Run Module or pressing F5 on your keyboard.
Creating variables, printing them, and doing other actions are called statements. Each statement ends with a newline. However you can write statements on the same line by putting a ; between them (not recommended).
You can combine strings and the values of variables in print statements by using a comma. For example:
print(name, "is", age, "years old.")
Commenting Code
- The # symbol is used to indicate the start of a comment.
Everything on a line following a # will be ignored by python.
This is useful for taking notes, documenting code, and indicating to others what your code is doing.
It is also used for temporarily removing unused code.
name = "John Smith" #This is a comment age = 12 #The comment will be ignored #Comments can be on their own lines #But each line must start with the correct symbol print(name) #print(age) Comments can be used to make python ignore #Code we don't want to delete. print(name, "is", age, "years old.")
Activity
- Create a new file and save it as learningVariables.py
- In the file create two new variables, one String variable and the other an Integer variable.
- Print them out to the console in a full sentence.
- Write comments to explain what your code does.