Turtle Module
This lesson will teach you how to import modules, use turtle, and debug code.
Importing Modules
We can add functionality to our programs by importing packages called modules.
A module is a file containing python definitions, statements, objects, and functions.
We will be using a module called Turtle to allow us to draw with code.
We can import turtle by typing this at the top of our file:
import turtleNOTE: Make sure when you name your file that you do not name it turtle.py otherwise you get a
Circular Import Error.
Once the module is imported, we can use an object variable to use the functions that came with it.
First, we need to create a Screen object to create a new drawing board, we'll call it window. Then we'll assign the Screen() function that came with the turtle module to our window object by using the = operator.
Next we'll make a Turtle object, which will allow us to draw on the drawing board. Let's call it pen. Similar to how we made the Screen object, we'll use the Turtle() function from the turtle module and assign them to pen by using =.
import turtle window = turtle.Screen() pen = turtle.Turtle()
Run your code and a new window will appear called Python Turtle Graphics.
In the center of the created window, you will see a little cursor. That cursor is used to draw graphics on the screen.
We can't draw yet. We have to give the cursor, or turtle, instructions on where to go. It's like a turtle drawing in the sand, as viewed from above.
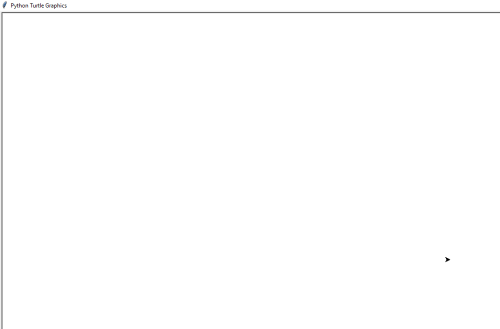
You can change the title of the window using the title() function of the Screen object.
import turtle window = turtle.Screen() pen = turtle.Turtle() window.title("Drawing Graphics")
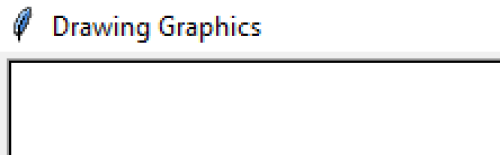
Turtle Functions
We still can't draw. We have to give the turtle instructions on where to go. The turtle object has functions that allow us to draw. These functions are simply named. For example, forward(), backward(), right(), left(), up(), down()
forward() and backward() take integers as arguments and move that many pixels in the given direction.
right() and left() also take integers as arguments and turn left or right by that many degrees.
up() and down() are not movement functions and will be explained later.
import turtle window = turtle.Screen() pen = turtle.Turtle() window.title("Drawing Graphics") pen.forward(300) pen.right(90) pen.forward(200)
The circle() function takes an integer as an argument and draws a circle with that number as the radius.
import turtle window = turtle.Screen() pen = turtle.Turtle() window.title("Drawing Graphics") pen.forward(300) pen.right(90) pen.forward(200) pen.circle(50)
The up() function takes no arguments and lifts the turtle (like lifting up a pencil from paper) to move around the screen without drawing anything.
The down() function places the turtle back down to resume drawing.
import turtle window = turtle.Screen() pen = turtle.Turtle() window.title("Drawing Graphics") pen.forward(300) pen.right(90) pen.forward(200) pen.circle(50) pen.up() pen.right(90) pen.forward(100) pen.down() pen.circle(50)
Challenge: Draw Shapes
- Create a new file and save it as learningTurtle.py
- Create a window and a turtle object.
- Rename the window to something else.
- Use the turtle functions to draw onto the window.
a. Draw a Square.
b. Draw a circle inside of a square.
Turtle Properties
You can use functions to change the look of the turtle lines.
pensize() takes an integer as an argument and makes the lines that many pixels thick.
color() takes a string as an argument and makes the lines that color.
import turtle window = turtle.Screen() pen = turtle.Turtle() window.title("Drawing Graphics") pen.forward(200) pen.pensize(20) pen.color("blue") pen.right(90) pen.forward(200)
Filling Shapes
A shape must be fully enclosed in order to be filled.
Start by setting the color to fill with using fillcolor(), which takes in a string argument.
Before drawing the shape, tell python you want to start filling by using begin_fill().
After the shape is completely drawn, tell python you're done using end_fill().
import turtle window = turtle.Screen() pen = turtle.Turtle() window.title("Drawing Graphics") #Sets fill color to blue and start filling pen.fillcolor("blue") pen.begin_fill() #Draws a square pen.forward(200) pen.right(90) pen.forward(200) pen.right(90) pen.forward(200) pen.right(90) pen.forward(200) #Ends filling pen.end_fill()
Challenge: Draw a Tree
- Create a new file and save it as project_tree.py
- Draw a brown rectangle for a trunk.
- Draw three or more circles for leaves on the tree.
a. Think about how to position your turtle while drawing.
b. Make sure to fill your shapes with color.
c. Remember to change the pen color to be the same as your fill color so there is no black outline.
You can find the complete list of turtle methods here. Keep in mind that these aren't necessary for this course, but you might find them interesting if you have extra time.
Set Position Function
Turtle has a function called setpos() (short for set position) that allows us to set its exact position on the screen.
The function takes two integer arguments, X and Y, that are used as coordinates.
The center of the window is (0, 0).
import turtle window = turtle.Screen() pen = turtle.Turtle() window.title("Set Position") pen.setpos(0, 100) pen.setpos(50, 100) pen.setpos(0, 0)
The setpos() function will move the turtle from its current location to the specified location, drawing a straight line between the points if the pen is down.
Debugging
When our code fails to run or doesn't work the way we want it to, it is said to have a bug.
Perfect code is rare and errors occur often, so you must get used to debugging.
Types of Errors and Methods for Debugging:
Syntax Errors are when you spell key words wrong or use them incorrectly. E.g. writing
beginfillinstead of
begin_fillwill give a syntax error.
You will get an error message when this happens, so it should be easy to debug. Check your spelling carefully.

Logic Errors occur when your program doesn't work as expected. This usually won't bring up an error message, so you have to go back through your code and change it once your realize your program isn't working right.
To avoid and debug logic errors, run your program every time you add a new feature (e.g draw a new shape) to make sure it works as expected. Temporarily commenting out code can also help with this.