Creating Collectibles and Hazards
In this tutorial, you will learn how to create more collectibles and hazards for your game.
More Collectibles
Time to add some more collectibles to the game. But to do that we need to change the FirstPersonController script to include a new collectible.
Find the FirstPersonController script inside of the Scripts folder, and double-click on it to open the script.
Once the script is open take a look at your code and compare it with the code below. Your code should look exactly the same at the top of the file except for the highlighted line.
To add another collectible, you need to add another line of code. Add the highlighted line to your code, save, and go back to Unity.
using System.Collections; using System.Collections.Generic; using UnityEngine; public class FirstPersonController : MonoBehaviour { public float xp = 0; public float coins = 0; public CharacterController controller; public float speed = 12f; public float gravity = -9.81f; public float jumpHeight = 3f; public Transform groundCheck; public float groundDistance = 0.4f; public LayerMask groundMask; Vector3 velocity; bool isGrounded;
After you save the script and return to Unity, you will see the new variable displayed in the FirstPersonController script attached to your Player object.
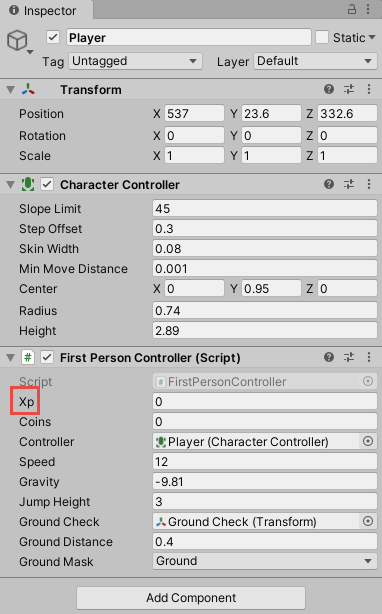
You can create as many variables as you want in the script, by copying everything else on the line for the
coinsvariable and adding a name for your new variable, like what we did with
xp.
Since we now have a new variable to work with, let's create a new script that will add to the XP variable when the player collects objects runs into the object.
In the Scripts folder, create a new script called
XPScript. Make sure you name it exactly
XPScript. Copy and paste the below code into the script.
using System.Collections; using System.Collections.Generic; using UnityEngine; public class XPScript : MonoBehaviour { public GameObject target; void Update() { //Moves towards the gameobject that is the target transform.position = Vector3.MoveTowards(transform.position, target.transform.position, .03f); } private void OnTriggerEnter(Collider other) { if (other.gameObject.tag == "Player") { other.GetComponent<FirstPersonController>().xp++; Destroy(gameObject); } } }
This script works a little differently than coins. The XP script has a variable of a Target, and will continually move towards that target object. In our game, the XP is going to continually move towards the player.
To add the new script, in the game, we need to create a new 3D Object. Create a sphere object inside of your scene, and change its size and position. Then, change the name of the object to
XP.
Make the object's Collider a Trigger, so that the player will be able to collect it. After that, add the XpScript to the object's Inspector just like you did for the CoinScript.
Now, we need to tell the script what the target is. Drag the game object Player into the script where it says Target. Now XP object will move towards the player.
Spike Hazard
Let's create some spike hazards for our game. If a player runs into the object, the level will restart.
Create a new script called
SpikeHazard, and copy the below code into that script.
using System.Collections; using System.Collections.Generic; using UnityEngine; public class SpikeHazard : MonoBehaviour { private void OnTriggerEnter(Collider other) { if (other.gameObject.tag == "Player") { //Reloads the level Application.LoadLevel(Application.loadedLevel); } } }
First, we need to create a 3d object that will act as the spike. A simple way to do this for now is to create a cube, and later replace that object with a real model of a spike.
Once you have your object in the scene, add the SpikeHazard script to its Inspector. Then make sure you check the Is Trigger box, to make the object a trigger.
Finally, this script works by checking to see if the object entering its trigger has the tag of
Player, so this tag will need to be added to our player object.
To add the
Playertag to the player object, select the Player object in the Hierarchy window to bring it up in the Inspector window. Click the Tag dropdown and select Player to add the player tag to the object.
When you touch the hazard object, the level will restart, and the player will have to play the level from the beginning.
Projectile Hazard
Now that we restart the level when the player runs into an obstacle, let's create an enemy that will shoot hazards. That way, the player will need to dodge them.
Create a script called
ProjectileHazard, and add the below code to the script.
using System.Collections; using System.Collections.Generic; using UnityEngine; public class ProjectileHazard : MonoBehaviour { //Detects the projectile and the speed public GameObject projectile; public float speed = 100f; //Creates a timer and sets a wait time private float timer; public int waitTime = 3; void Update() { //The timer waits for 3 seconds then "shoots" the projectile timer += Time.deltaTime; if(timer > waitTime) { //calls the "shoot" method shoot(); timer = 0; } } //Defines the method "shoot" where the rigidbody of the object is used to move the projectile void shoot() { GameObject bullet = Instantiate(projectile, transform.position, Quaternion.identity) as GameObject; bullet.AddComponent<Rigidbody>(); Rigidbody projectileBody = bullet.GetComponent<Rigidbody>(); projectileBody.AddForce(Vector3.forward* speed); //Destroys the projectile after 1 second Destroy(bullet, 1.0f); } }
Create an Empty Game Object, call it
Emitter, and place it somewhere in the scene. In the object's Inspector add the ProjectileHazard script. Drag the Hazard game object into the script, so it knows that it should fire the Hazard object. Then, adjust the speed and wait time as much as you like.
You can also reduce the Wait Time to cause the hazards to shoot out quicker.
Make your new hazard into a prefab, and place it around your map! Try adjusting its color, or creating different Hazard emitters that fire at different speeds or have different wait times.